#include<stdlib.h>
int main()
{
system("C:\\Windows\\System32\\ipconfig");
return 0;
}
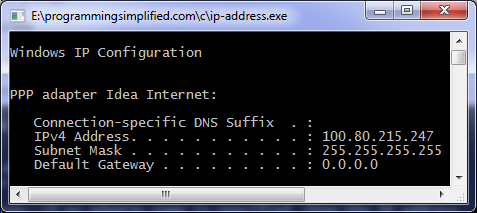
int main()
{
system("C:\\Windows\\System32\\ipconfig");
return 0;
}
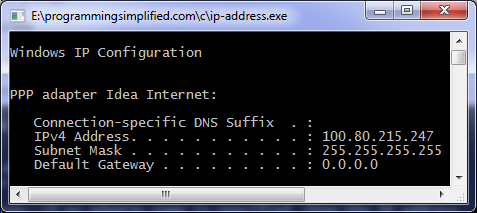
great place for C programmers
/* Write a C program to illustrate the concept of unions*/#include <stdio.h> #include <conio.h>
/* Write a C program to illustrate the operations of singly linked list */#include <stdio.h> #include <stdlib.h> #include <conio.h> #include <string.h> #define MAX 30
/* Write a C program to implement stack. Stack is a LIFO data strcuture * * LIFO - Last in First Out * * Perform PUSH(insert operation), POP(Delete operation) and Display stack */#include <stdio.h> #include <conio.h> #define MAXSIZE 5
#include<stdio.h> int main( void ){ int year = 2012; if( year % 4 == 0 && year % 100 != 0 ) { printf("Leap Year..\n"); }else if (year % 4 == 0 && year % 400 == 0 ){ printf("Leap Year..\n"); }else{ printf("Not Leap Year..\n"); } return 0; }
#include<stdio.h> int main( void ){ int year = 2012; if(( year % 4 == 0 && year % 100 != 0 ) || (year % 4 == 0 && year % 400 == 0 )){ printf("Leap Year..\n"); }else{ printf("Not Leap Year..\n"); } return 0; }
printf("Hello, World! \n");
/0
. For example:"c string tutorial"
char
type.char s[5];
// inline_functions_macro.c #include <stdio.h> #include <conio.h> #define toupper(a) ((a) >= 'a' && ((a) <= 'z') ? ((a)-('a'-'A')):(a)) int main() { char ch; printf("Enter a character: "); ch = toupper( getc(stdin) ); printf( "%c", ch ); }
Sample Input: xyz Sample Output: Z
// inline_functions_inline.cpp #include <stdio.h> #include <conio.h> inline char toupper( char a ) { return ((a >= 'a' && a <= 'z') ? a-('a'-'A') : a ); } int main() { printf("Enter a character: "); char ch = toupper( getc(stdin) ); printf( "%c", ch ); }
Sample Input: a Sample Output: A