C Programming Pointers
Pointers are the powerful feature of C and (C++) programming, which differs it from other popular programming languages like: java and Visual Basic.
Pointers are used in C program to access the memory and manipulate the address.
Reference operator(&)
If var is a variable then,
&var
is the address in memory.
/* Example to demonstrate use of reference operator in C programming. */
#include <stdio.h>
int main(){
int var=5;
printf("Value: %d\n",var);
printf("Address: %d",&var); //Notice, the ampersand(&) before var.
return 0;
}
Output
Value: 5 Address: 2686778
Note: You may obtain different value of address while using this code.
In above source code, value 5 is stored in the memory location 2686778. var is just the name given to that location.
You, have already used reference operator in C program while using
scanf()
function.scanf("%d",&var);
Reference operator(*) and Pointer variables
Pointers variables are the special types of variables that holds memory address rather than data, that is, a variable that holds address value is called a pointer variable or simply a pointer.
Declaration of Pointer
Dereference operator(*) are used to identify an operator as a pointer.
data_type * pointer_variable_name; int *p;
Above statement defines, p as pointer variable of type int.
Example To Demonstrate Working of Pointers
/* Source code to demonstrate, handling of pointers in C program */
#include <stdio.h>
int main(){
int *pc,c;
c=22;
printf("Address of c:%d\n",&c);
printf("Value of c:%d\n\n",c);
pc=&c;
printf("Address of pointer pc:%d\n",pc);
printf("Content of pointer pc:%d\n\n",*pc);
c=11;
printf("Address of pointer pc:%d\n",pc);
printf("Content of pointer pc:%d\n\n",*pc);
*pc=2;
printf("Address of c:%d\n",&c);
printf("Value of c:%d\n\n",c);
return 0;
}
Output
Address of c: 2686784 Value of c: 22 Address of pointer pc: 2686784 Content of pointer pc: 22 Address of pointer pc: 2686784 Content of pointer pc: 11 Address of c: 2686784 Value of c: 2

Explanation of program and figure
- Code
int *pc, p;
creates a pointer pc and a variable c. Pointer pc points to some address and that address has garbage value. Similarly, variable c also has garbage value at this point. - Code
c=22;
makes the value of c equal to 22, i.e.,22 is stored in the memory location of variablec. - Code pc=&c; makes pointer, point to address of c. Note that,
&c
is the address of variablec (because c is normal variable) and pc is the address of pc (because pc is the pointer variable). Since the address of pc and address of c is same,*pc
(value of pointer pc) will be equal to the value of c. - Code
c=11;
makes the value of c, 11. Since, pointer pc is pointing to address of c. Value of *pcwill also be 11. - Code
*pc=2;
change the contents of the memory location pointed by pointer pc to change to 2. Since address of pointer pc is same as address of c, value of c also changes to 2.
Commonly done mistakes in pointers
Suppose, the programmer want pointer pc to point to the address of c. Then,
int c, *pc; pc=c; /* pc is address whereas, c is not an address. */ *pc=&c; /* &c is address whereas, *pc is not an address. */
In both cases, pointer pc is not pointing to the address of c.
C Programming Pointers and Arrays
Arrays are closely related to pointers in C programming but the important difference between them is that, a pointer variable can take different addresses as value whereas, in case of array it is fixed. This can be demonstrated by an example:
#include <stdio.h>
int main(){
char c[4];
int i;
for(i=0;i<4;++i){
printf("Address of c[%d]=%x\n",i,&c[i]);
}
return 0;
}
Address of c[0]=28ff44 Address of c[1]=28ff45 Address of c[2]=28ff46 Address of c[3]=28ff47
Notice, that there is equal difference (difference of 1 byte) between any two consecutive elements of array.
Note: You may get different address of an array.
Relation between Arrays and Pointers
Consider and array:
int arr[4];
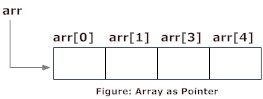
In arrays of C programming, name of the array always points to the first element of an array. Here, address of first element of an array is
&arr[0]
. Also, arr represents the address of the pointer where it is pointing. Hence, &arr[0] is equivalent to
arr.
Also, value inside the address
&arr[0]
and address arr are equal. Value in address &arr[0]
isarr[0]
and value in address arr is *arr
. Hence, arr[0] is equivalent to *arr
.
Similarly,
&a[1] is equivalent to (a+1) AND, a[1] is equivalent to *(a+1). &a[2] is equivalent to (a+2) AND, a[2] is equivalent to *(a+2). &a[3] is equivalent to (a+1) AND, a[3] is equivalent to *(a+3). . . &a[i] is equivalent to (a+i) AND, a[i] is equivalent to *(a+i).
In C, you can declare an array and can use pointer to alter the data of an array.
//Program to find the sum of six numbers with arrays and pointers.
#include <stdio.h>
int main(){
int i,class[6],sum=0;
printf("Enter 6 numbers:\n");
for(i=0;i<6;++i){
scanf("%d",(class+i)); // (class+i) is equivalent to &class[i]
sum += *(class+i); // *(class+i) is equivalent to class[i]
}
printf("Sum=%d",sum);
return 0;
}
Output
Enter 6 numbers:
2
3
4
5
3
4
Sum=21
C Programming Pointers and Functions - Call by Reference
When, argument is passed using pointer, address of the memory location is passed instead of value.
Example of Pointer And Functions
Program to swap two number using call by reference.
/* C Program to swap two numbers using pointers and function. */
#include <stdio.h>
void swap(int *a,int *b);
int main(){
int num1=5,num2=10;
swap(&num1,&num2); /* address of num1 and num2 is passed to swap function */
printf("Number1 = %d\n",num1);
printf("Number2 = %d",num2);
return 0;
}
void swap(int *a,int *b){ /* pointer a and b points to address of num1 and num2 respectively */
int temp;
temp=*a;
*a=*b;
*b=temp;
}
Output
Number1 = 10 Number2 = 5
Explanation
The address of memory location num1 and num2 are passed to function and the pointers
*a
and *b
accept those values. So, the pointer a and b points to address of num1 and num2 respectively. When, the value of pointer are changed, the value in memory location also changed correspondingly. Hence, change made to *a
and *b
was reflected in num1 and num2 in main function.
This technique is known as call by reference in C programming.
C Programming Dynamic Memory Allocation
The exact size of array is unknown untill the compile time,i.e., time when a compier compiles code written in a programming language into a executable form. The size of array you have declared initially can be sometimes insufficient and sometimes more than required. Dynamic memory allocation allows a program to obtain more memory space, while running or to release space when no space is required.
Although, C language inherently does not has any technique to allocated memory dynamically, there are 4 library functions under
"stdlib.h"
for dynamic memory allocation.Function | Use of Function |
---|---|
malloc() | Allocates requested size of bytes and returns a pointer first byte of allocated space |
calloc() | Allocates space for an array elements, initializes to zero and then returns a pointer to memory |
free() | dellocate the previously allocated space |
realloc() | Change the size of previously allocated space |
malloc()
The name malloc stands for "memory allocation". The function
malloc()
reserves a block of memory of specified size and return a pointer of type void
which can be casted into pointer of any form.Syntax of malloc()
ptr=(cast-type*)malloc(byte-size)
Here, ptr is pointer of cast-type. The
malloc()
function returns a pointer to an area of memory with size of byte size. If the space is insufficient, allocation fails and returns NULL pointer.ptr=(int*)malloc(100*sizeof(int));
This statement will allocate either 200 or 400 according to size of
int
2 or 4 bytes respectively and the pointer points to the address of first byte of memory.calloc()
The name calloc stands for "contiguous allocation". The only difference between malloc() and calloc() is that, malloc() allocates single block of memory whereas calloc() allocates multiple blocks of memory each of same size and sets all bytes to zero.
Syntax of calloc()
ptr=(cast-type*)calloc(n,element-size);
This statement will allocate contiguous space in memory for an array of n elements. For example:
ptr=(float*)calloc(25,sizeof(float));
This statement allocates contiguous space in memory for an array of 25 elements each of size of float, i.e, 4 bytes.
free()
Dynamically allocated memory with either calloc() or malloc() does not get return on its own. The programmer must use free() explicitly to release space.
syntax of free()
free(ptr);
This statement cause the space in memory pointer by ptr to be deallocated.
Examples of calloc() and malloc()
Write a C program to find sum of n elements entered by user. To perform this program, allocate memory dynamically using malloc() function.
#include <stdio.h>
#include <stdlib.h>
int main(){
int n,i,*ptr,sum=0;
printf("Enter number of elements: ");
scanf("%d",&n);
ptr=(int*)malloc(n*sizeof(int)); //memory allocated using malloc
if(ptr==NULL)
{
printf("Error! memory not allocated.");
exit(0);
}
printf("Enter elements of array: ");
for(i=0;i<n;++i)
{
scanf("%d",ptr+i);
sum+=*(ptr+i);
}
printf("Sum=%d",sum);
free(ptr);
return 0;
}
Write a C program to find sum of n elements entered by user. To perform this program, allocate memory dynamically using calloc() function.
#include <stdio.h>
#include <stdlib.h>
int main(){
int n,i,*ptr,sum=0;
printf("Enter number of elements: ");
scanf("%d",&n);
ptr=(int*)calloc(n,sizeof(int));
if(ptr==NULL)
{
printf("Error! memory not allocated.");
exit(0);
}
printf("Enter elements of array: ");
for(i=0;i<n;++i)
{
scanf("%d",ptr+i);
sum+=*(ptr+i);
}
printf("Sum=%d",sum);
free(ptr);
return 0;
}
realloc()
If the previously allocated memory is insufficient or more than sufficient. Then, you can change memory size previously allocated using realloc().
Syntax of realloc()
ptr=realloc(ptr,newsize);
Here, ptr is reallocated with size of newsize.
#include <stdio.h>
#include <stdlib.h>
int main(){
int *ptr,i,n1,n2;
printf("Enter size of array: ");
scanf("%d",&n1);
ptr=(int*)malloc(n1*sizeof(int));
printf("Address of previously allocated memory: ");
for(i=0;i<n1;++i)
printf("%u\t",ptr+i);
printf("\nEnter new size of array: ");
scanf("%d",&n2);
ptr=realloc(ptr,n2);
for(i=0;i<n2;++i)
printf("%u\t",ptr+i);
return 0;
}
No comments:
Post a Comment