C Programming Functions
In programming, a function is a segment that groups code to perform a specific task.
A C program has at least one function
main( )
. Without main()
function, there is technically no C program.Types of C functions
Basically, there are two types of functions in C on basis of whether it is defined by user or not.
- Library function
- User defined function
Library function
Library functions are the in-built function in C programming system. For example:
main()
- The execution of every C program starts from this
main()
function.printf()
-
prinf()
is used for displaying output in C.scanf()
-
scanf()
is used for taking input in C.
Visit this page to learn more about library functions in C programming language.
User defined function
C allows programmer to define their own function according to their requirement. These types of functions are known as user-defined functions. Suppose, a programmer wants to find factorial of a number and check whether it is prime or not in same program. Then, he/she can create two separate user-defined functions in that program: one for finding factorial and other for checking whether it is prime or not.
How user-defined function works in C Programming?
#include <stdio.h> void function_name(){ ................ ................ } int main(){ ........... ........... function_name(); ........... ........... }
As mentioned earlier, every C program begins from
main()
and program starts executing the codes inside main()
function. When the control of program reaches to function_name()
inside main()
function. The control of program jumps to void function_name()
and executes the codes inside it. When all the codes inside that user-defined function are executed, control of the program jumps to the statement just after function_name()
from where it is called. Analyze the figure below for understanding the concept of function in C programming. Visit this page to learn in detail about user-defined functions.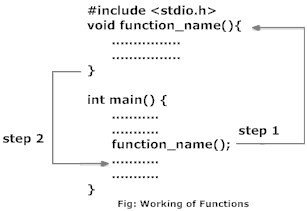
Remember, the function name is an identifier and should be unique.
Advantages of user defined functions
- User defined functions helps to decompose the large program into small segments which makes programmar easy to understand, maintain and debug.
- If repeated code occurs in a program. Function can be used to include those codes and execute when needed by calling that function.
- Programmer working on large project can divide the workload by making different functions.
C Programming User-defined functions
This chapter is the continuation to the function Introduction chapter.
Example of user-defined function
Write a C program to add two integers. Make a function
add
to add integers and display sum inmain()
function./*Program to demonstrate the working of user defined function*/
#include <stdio.h>
int add(int a, int b); //function prototype(declaration)
int main(){
int num1,num2,sum;
printf("Enters two number to add\n");
scanf("%d %d",&num1,&num2);
sum=add(num1,num2); //function call
printf("sum=%d",sum);
return 0;
}
int add(int a,int b) //function declarator
{
/* Start of function definition. */
int add;
add=a+b;
return add; //return statement of function
/* End of function definition. */
}
Function prototype(declaration):
Every function in C programming should be declared before they are used. These type of declaration are also called function prototype. Function prototype gives compiler information about function name, type of arguments to be passed and return type.
Syntax of function prototype
return_type function_name(type(1) argument(1),....,type(n) argument(n));
In the above example,
int add(int a, int b);
is a function prototype which provides following information to the compiler:- name of the function is
add()
- return type of the function is
int
. - two arguments of type
int
are passed to function.
Function prototype are not needed if user-definition function is written before
main()
function.Function call
Control of the program cannot be transferred to user-defined function unless it is called invoked.
Syntax of function call
function_name(argument(1),....argument(n));
In the above example, function call is made using statement
add(num1,num2);
from main()
. This make the control of program jump from that statement to function definition and executes the codes inside that function.Function definition
Function definition contains programming codes to perform specific task.
Syntax of function definition
return_type function_name(type(1) argument(1),..,type(n) argument(n)) { //body of function }
Function definition has two major components:
1. Function declarator
Function declarator is the first line of function definition. When a function is called, control of the program is transferred to function declarator.
Syntax of function declarator
return_type function_name(type(1) argument(1),....,type(n) argument(n))
Syntax of function declaration and declarator are almost same except, there is no semicolon at the end of declarator and function declarator is followed by function body.
In above example,
int add(int a,int b)
in line 12 is a function declarator.2. Function body
Function declarator is followed by body of function inside braces.
Passing arguments to functions
In programming, argument(parameter) refers to data this is passed to function(function definition) while calling function.
In above example two variable, num1 and num2 are passed to function during function call and these arguments are accepted by arguments a and b in function definition.

Arguments that are passed in function call and arguments that are accepted in function definition should have same data type. For example:
If argument num1 was of int type and num2 was of float type then, argument variable a should be of type int and b should be of type float,i.e., type of argument during function call and function definition should be same.
A function can be called with or without an argument.
Return Statement
Return statement is used for returning a value from function definition to calling function.
Syntax of return statement
return (expression);
For example:
return a; return (a+b);
In above example, value of variable add in
add()
function is returned and that value is stored in variable sum in main()
function. The data type of expression in return statement should also match the return type of function.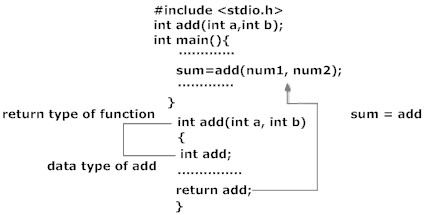
Types of User-defined Functions in C Programming
For better understanding of arguments and return type in functions, user-defined functions can be categorised as:
- Function with no arguments and no return value
- Function with no arguments and return value
- Function with arguments but no return value
- Function with arguments and return value.
Let's take an example to find whether a number is prime or not using above 4 cateogories of user defined functions.
Function with no arguments and no return value.
/*C program to check whether a number entered by user is prime or not using function with no arguments and no return value*/
#include <stdio.h>
void prime();
int main(){
prime(); //No argument is passed to prime().
return 0;
}
void prime(){
/* There is no return value to calling function main(). Hence, return type of prime() is void */
int num,i,flag=0;
printf("Enter positive integer enter to check:\n");
scanf("%d",&num);
for(i=2;i<=num/2;++i){
if(num%i==0){
flag=1;
}
}
if (flag==1)
printf("%d is not prime",num);
else
printf("%d is prime",num);
}
Function
prime()
is used for asking user a input, check for whether it is prime of not and display it accordingly. No argument is passed and returned form prime()
function.Function with no arguments but return value
/*C program to check whether a number entered by user is prime or not using function with no arguments but having return value */
#include <stdio.h>
int input();
int main(){
int num,i,flag = 0;
num=input(); /* No argument is passed to input() */
for(i=2; i<=num/2; ++i){
if(num%i==0){
flag = 1;
break;
}
}
if(flag == 1)
printf("%d is not prime",num);
else
printf("%d is prime", num);
return 0;
}
int input(){ /* Integer value is returned from input() to calling function */
int n;
printf("Enter positive integer to check:\n");
scanf("%d",&n);
return n;
}
There is no argument passed to
input()
function But, the value of n is returned from input()
tomain()
function.Function with arguments and no return value
/*Program to check whether a number entered by user is prime or not using function with arguments and no return value */
#include <stdio.h>
void check_display(int n);
int main(){
int num;
printf("Enter positive enter to check:\n");
scanf("%d",&num);
check_display(num); /* Argument num is passed to function. */
return 0;
}
void check_display(int n){
/* There is no return value to calling function. Hence, return type of function is void. */
int i, flag = 0;
for(i=2; i<=n/2; ++i){
if(n%i==0){
flag = 1;
break;
}
}
if(flag == 1)
printf("%d is not prime",n);
else
printf("%d is prime", n);
}
Here,
check_display()
function is used for check whether it is prime or not and display it accordingly. Here, argument is passed to user-defined function but, value is not returned from it to calling function.Function with argument and a return value
/* Program to check whether a number entered by user is prime or not using function with argument and return value */
#include <stdio.h>
int check(int n);
int main(){
int num,num_check=0;
printf("Enter positive enter to check:\n");
scanf("%d",&num);
num_check=check(num); /* Argument num is passed to check() function. */
if(num_check==1)
printf("%d is not prime",num);
else
printf("%d is prime",num);
return 0;
}
int check(int n){
/* Integer value is returned from function check() */
int i;
for(i=2;i<=n/2;++i){
if(n%i==0)
return 1;
}
return 0;
}
Here,
check()
function is used for checking whether a number is prime or not. In this program, input from user is passed to function check()
and integer value is returned from it. If input the number is prime, 0 is returned and if number is not prime, 1 is returned.C Programming Recursion
A function that calls itself is known as recursive function and this technique is known as recursion in C programming.
Example of recursion in C programming
Write a C program to find sum of first n natural numbers using recursion. Note: Positive integers are known as natural number i.e. 1, 2, 3....n
#include <stdio.h>
int sum(int n);
int main(){
int num,add;
printf("Enter a positive integer:\n");
scanf("%d",&num);
add=sum(num);
printf("sum=%d",add);
}
int sum(int n){
if(n==0)
return n;
else
return n+sum(n-1); /*self call to function sum() */
}
Output
Enter a positive integer: 5 15
In, this simple C program,
sum()
function is invoked from the same function. If n is not equal to 0 then, the function calls itself passing argument 1 less than the previous argument it was called with. Suppose, n is 5 initially. Then, during next function calls, 4 is passed to function and the value of argument decreases by 1 in each recursive call. When, n becomes equal to 0, the value of n is returned which is the sum numbers from 5 to 1.
For better visualization of recursion in this example:
sum(5) =5+sum(4) =5+4+sum(3) =5+4+3+sum(2) =5+4+3+2+sum(1) =5+4+3+2+1+sum(0) =5+4+3+2+1+0 =5+4+3+2+1 =5+4+3+3 =5+4+6 =5+10 =15
Every recursive function must be provided with a way to end the recursion. In this example when, nis equal to 0, there is no recursive call and recursion ends.
Advantages and Disadvantages of Recursion
Recursion is more elegant and requires few variables which make program clean. Recursion can be used to replace complex nesting code by dividing the problem into same problem of its sub-type.
In other hand, it is hard to think the logic of a recursive function. It is also difficult to debug the code containing recursion.
C Programming Storage Class
Every variable in C programming has two properties: type and storage class. Type refers to the data type of variable whether it is character or integer or floating-point value etc. And storage class determines how long it stays in existence.
There are 4 types of storage class:
- automatic
- external
- static
- register
Automatic storage class
Keyword for automatic variable
auto
Variables declared inside the function body are automatic by default. These variable are also known as local variables as they are local to the function and doesn't have meaning outside that function
Since, variable inside a function is automatic by default, keyword auto are rarely used.
External storage class
External variable can be accessed by any function. They are also known as global variables. Variables declared outside every function are external variables.
In case of large program, containing more than one file, if the global variable is declared in file 1 and that variable is used in file 2 then, compiler will show error. To solve this problem, keyword
extern
is used in file 2 to indicate that, the variable specified is global variable and declared in another file.Example to demonstrate working of external variable
#include
void Check();
int a=5;
/* a is global variable because it is outside every function */
int main(){
a+=4;
Check();
return 0;
}
void Check(){
++a;
/* ----- Variable a is not declared in this function but, works in any function as they are global variable ------- */
printf("a=%d\n",a);
}
Output
a=10
Register Storage Class
Keyword to declare register variable
register
Example of register variable
register int a;
Register variables are similar to automatic variable and exists inside that particular function only.
If the compiler encounters register variable, it tries to store variable in microprocessor's register rather than memory. Value stored in register are much faster than that of memory.
In case of larger program, variables that are used in loops and function parameters are declared register variables.
Since, there are limited number of register in processor and if it couldn't store the variable in register, it will automatically store it in memory.
Static Storage Class
The value of static variable persists until the end of the program. A variable can be declared static using keyword:
static
. For example:static int i;
Here, i is a static variable.
Example to demonstrate the static variable
#include <stdio.h>
void Check();
int main(){
Check();
Check();
Check();
}
void Check(){
static int c=0;
printf("%d\t",c);
c+=5;
}
Output
0 5 10
During first function call, it will display 0. Then, during second function call, variable c will not be initialized to 0 again, as it is static variable. So, 5 is displayed in second function call and 10 in third call.
If variable c had been automatic variable, the output would have been:
0 0 0
Thanks nice article. Here I found the description of how function works
ReplyDeletehttp://www.techcrashcourse.com/2015/05/c-programming-language-functions.html