C Programming Arrays
In C programming, one of the frequently arising problem is to handle similar types of data. For example: If the user want to store marks of 100 students. This can be done by creating 100 variable individually but, this process is rather tedious and impracticable. These type of problem can be handled in C programming using arrays.
An array is a sequence of data item of homogeneous value(same type).
Arrays are of two types:
- One-dimensional arrays
- Multidimensional arrays( will be discussed in next chapter )
Declaration of one-dimensional array
data_type array_name[array_size]; For example: int age[5];
Here, the name of array is age. The size of array is 5,i.e., there are 5 items(elements) of array age. All element in an array are of the same type (int, in this case).
Array elements
Size of array defines the number of elements in an array. Each element of array can be accessed and used by user according to the need of program. For example:
int age[5];
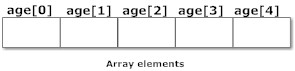
Note that, the first element is numbered 0 and so on.
Here, the size of array age is 5 times the size of int because there are 5 elements.
Suppose, the starting address of
age[0]
is 2120d and the size of int be 4 bytes. Then, the next address (address of a[1]
) will be 2124d, address of a[2]
will be 2128d and so on.Initialization of one-dimensional array:
Arrays can be initialized at declaration time in this source code as:
int age[5]={2,4,34,3,4};
It is not necessary to define the size of arrays during initialization.
int age[]={2,4,34,3,4};
In this case, the compiler determines the size of array by calculating the number of elements of an array.
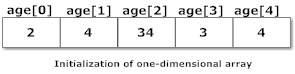
Accessing array elements
In C programming, arrays can be accessed and treated like variables in C.
For example:
scanf("%d",&age[2]); /* statement to insert value in the third element of array age[]. */ scanf("%d",&age[i]); /* Statement to insert value in (i+1)th element of array age[]. */ /* Because, the first element of array is age[0], second is age[1], ith is age[i-1] and (i+1)th is age[i]. */ printf("%d",age[0]); /* statement to print first element of an array. */ printf("%d",age[i]); /* statement to print (i+1)th element of an array. */
Example of array in C programming
/* C program to find the sum marks of n students using arrays */
#include <stdio.h>
int main(){
int marks[10],i,n,sum=0;
printf("Enter number of students: ");
scanf("%d",&n);
for(i=0;i<n;++i){
printf("Enter marks of student%d: ",i+1);
scanf("%d",&marks[i]);
sum+=marks[i];
}
printf("Sum= %d",sum);
return 0;
}
Output
Enter number of students: 3 Enter marks of student1: 12 Enter marks of student2: 31 Enter marks of student3: 2 sum=45
Important thing to remember in C arrays
Suppose, you declared the array of 10 students. For example:
arr[10]
. You can use array members from arr[0]
to arr[9]
. But, what if you want to use element arr[10]
, arr[13]
etc. Compiler may not show error using these elements but, may cause fatal error during program execution.C Programming Multidimensional Arrays
C programming language allows programmer to create arrays of arrays known as multidimensional arrays. For example:
float a[2][6];
Here, a is an array of two dimension, which is an example of multidimensional array. This array has 2 rows and 6 columns
For better understanding of multidimensional arrays, array elements of above example can be thinked of as below:
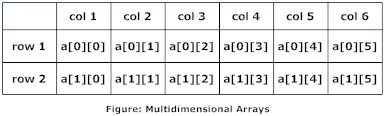
Initialization of Multidimensional Arrays
In C, multidimensional arrays can be initialized in different number of ways.
int c[2][3]={{1,3,0}, {-1,5,9}}; OR int c[][3]={{1,3,0}, {-1,5,9}}; OR int c[2][3]={1,3,0,-1,5,9};
Initialization Of three-dimensional Array
double cprogram[3][2][4]={ {{-0.1, 0.22, 0.3, 4.3}, {2.3, 4.7, -0.9, 2}}, {{0.9, 3.6, 4.5, 4}, {1.2, 2.4, 0.22, -1}}, {{8.2, 3.12, 34.2, 0.1}, {2.1, 3.2, 4.3, -2.0}} };
Suppose there is a multidimensional array
arr[i][j][k][m]
. Then this array can hold i*j*k*m numbers of data.
Similarly, the array of any dimension can be initialized in C programming.
Example of Multidimensional Array In C
Write a C program to find sum of two matrix of order 2*2 using multidimensional arrays where, elements of matrix are entered by user.
#include <stdio.h>
int main(){
float a[2][2], b[2][2], c[2][2];
int i,j;
printf("Enter the elements of 1st matrix\n");
/* Reading two dimensional Array with the help of two for loop. If there was an array of 'n' dimension, 'n' numbers of loops are needed for inserting data to array.*/
for(i=0;i<2;++i)
for(j=0;j<2;++j){
printf("Enter a%d%d: ",i+1,j+1);
scanf("%f",&a[i][j]);
}
printf("Enter the elements of 2nd matrix\n");
for(i=0;i<2;++i)
for(j=0;j<2;++j){
printf("Enter b%d%d: ",i+1,j+1);
scanf("%f",&b[i][j]);
}
for(i=0;i<2;++i)
for(j=0;j<2;++j){
/* Writing the elements of multidimensional array using loop. */
c[i][j]=a[i][j]+b[i][j]; /* Sum of corresponding elements of two arrays. */
}
printf("\nSum Of Matrix:");
for(i=0;i<2;++i)
for(j=0;j<2;++j){
printf("%.1f\t",c[i][j]);
if(j==1) /* To display matrix sum in order. */
printf("\n");
}
return 0;
}
Ouput
Enter the elements of 1st matrix Enter a11: 2; Enter a12: 0.5; Enter a21: -1.1; Enter a22: 2; Enter the elements of 2nd matrix Enter b11: 0.2; Enter b12: 0; Enter b21: 0.23; Enter b22: 23; Sum Of Matrix: 2.2 0.5 -0.9 25.0
C Programming Arrays and Functions
In C programming, a single array element or an entire array can be passed to a function. Also, both one-dimensional and multi-dimensional array can be passed to function as argument.
Passing One-dimensional Array In Function
C program to pass a single element of an array to function
#include <stdio.h>
void display(int a)
{
printf("%d",a);
}
int main(){
int c[]={2,3,4};
display(c[2]); //Passing array element c[2] only.
return 0;
}
Output
4
Single element of an array can be passed in similar manner as passing variable to a function.
Passing entire one-dimensional array to a function
While passing arrays to the argument, the name of the array is passed as an argument(,i.e, starting address of memory area is passed as argument).
Write a C program to pass an array containing age of person to a function. This function should find average age and display the average age in main function.
#include <stdio.h>
float average(float a[]);
int main(){
float avg, c[]={23.4, 55, 22.6, 3, 40.5, 18};
avg=average(c); /* Only name of array is passed as argument. */
printf("Average age=%.2f",avg);
return 0;
}
float average(float a[]){
int i;
float avg, sum=0.0;
for(i=0;i<6;++i){
sum+=a[i];
}
avg =(sum/6);
return avg;
}
Output
Average age=27.08
Passing Multi-dimensional Arrays to Function
To pass two-dimensional array to a function as an argument, starting address of memory area reserved is passed as in one dimensional array
Example to pass two-dimensional arrays to function
#include
void Function(int c[2][2]);
int main(){
int c[2][2],i,j;
printf("Enter 4 numbers:\n");
for(i=0;i<2;++i)
for(j=0;j<2;++j){
scanf("%d",&c[i][j]);
}
Function(c); /* passing multi-dimensional array to function */
return 0;
}
void Function(int c[2][2]){
/* Instead to above line, void Function(int c[][2]){ is also valid */
int i,j;
printf("Displaying:\n");
for(i=0;i<2;++i)
for(j=0;j<2;++j)
printf("%d\n",c[i][j]);
}
Output
Enter 4 numbers: 2 3 4 5 Displaying: 2 3 4 5
Arrays in C in details
ReplyDeleteVery Very Thanks nice article multidimensional array
ReplyDelete